In this article, we will see how to write a python program to check Leap Year. A year is said to be a leap year if it’s completely divided by 4. In other words, leap year always gives a reminder of zero after dividing by four. But these conditions are not satisfied for the century years.
Century Years: Century years that end with 00 like 1600, 1700, 1800, 1900, 2000, etc.
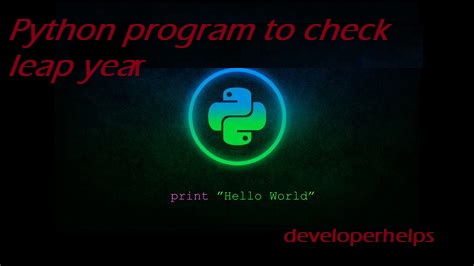
A century year is a leap year if it is completely divisibly by 400. You should have a basic knowledge of Python Operators.
For example, The year 2004 is a leap year because it’s completely divisible by 4. Whereas 2013 is not a leap year because its last two digits 13 are not divisible by 4 completely.
So, our problem to check whether a year is a leap year or not is solved into three parts:
Check whether a year is divisible by 4 or not. if it is divisible by 4 then it’s a leap year
And if it is not divisible by 4 then check if it’s divided by 400 because maybe the year will be a century year
And if it is not divisible by 400 that means this is not a leap year because neither it divides by 4 nor 400.
Note: A century year is always divided by 100 whereas a normal year doesn’t divide by 100.
Python Program to Check Leap Year
print("Enter a year")
n = int(input())
#For a normal year
if(n%4==0 and n%100!=0):
print("leap year(normal year)")
#For a century year
elif(n%400==0 and n%100==0):
print("leap year (century year)")
else:
print("Not leap year")
Output (First Run):
Enter a year
1900
Not leap year
Output (Second Run):
Enter a year
2000
leap year (century year)
Output (Third Run):
Enter a year
1996
leap year(normal year)