Python try except and the block is to test a particular block of code to make sure if it runs properly before the rest of the program runs. You have written a big new code for a program, you want to ensure it runs before letting the rest of the program run. tryβ¦except block allows running your code and manage an exception if the user detects one.
Add finally and else statements to process extra code depending on the result of the tryβ¦except block. The Python tryβ¦except statement interprets the code under the βtryβ section. If the code does not execute, the program will stop where it detected the error, and the βexceptβ will run. The try block provides you to process a block of code for mistakes. The except block allows you to manage the error with a response. The syntax for the tryβ¦except block:
try:
βyour code…
except:
βyour code…
Exception Handling
The try and except block are to detect and manage exceptions. Python runs a code regarding the try statement as a part of the program. The except statement works as the programβs answer to any exceptions in the previous try clause.
Exceptions are easy to handle errors and particular conditions in a program, if you are executing a code that results in an error then use exception handling. Moreover, you can alert an exception in your program using the raise exception statement. Detecting an exception deintegrates the current code running and falls back to the exception back until it is operated.
Example :
amount = 9000
# check that You are eligible to
# purchase Dsa Self Paced or not
if(amount > 2999)
print("You are eligible to purchase Dsa Self Paced")
Output :
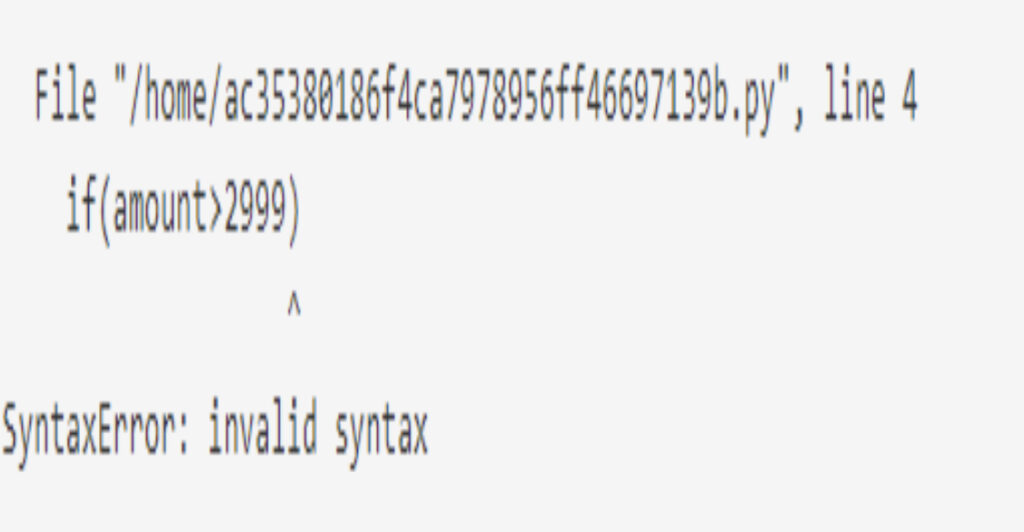
The syntax error here is due to the wrong syntax in the code. It leads to the termination of the program.
Let us now see how the user can handle this exception. Check out the code below:
Example :
marks = 10000
a = marks / 0
print(a)
Output :
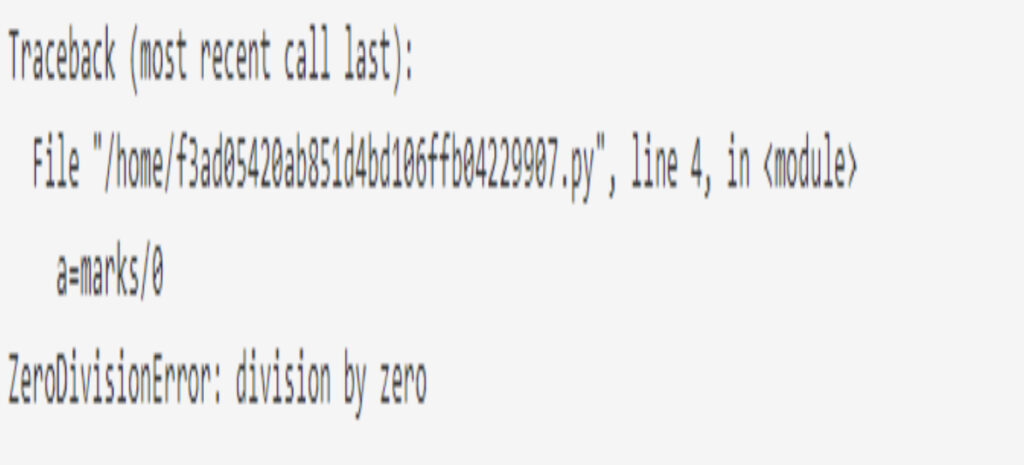
Multiple Except Statements
You can reiterate except statements for many types of errors to detect for multiple exceptions. This is better if you think that one of the exceptions may be alert but you are unsure which one you will face. An illustration of tryβ¦except blocks that search for a Name Error:
Example :
try:
βprint(our variable)
except NameError:
βprint('ourVariable is not defined')
except:
βprint('Error returned')
Here, the code that sends back our Variable is not described because the code returns a Name Error. We can increase the errors, such as an OSError or a Zero Division Error.
Python Finally Block
If you want to message print in both cases where an error is returned and if no error is detected. This happens when the final block executes. If you run a finally clause, its composition will be run regardless of if they tryβ¦except block detects an error. Finally, restrictions are indicators that your code has been executed, because they are not distinctive from a code that has been executed, they are not as generally executed.
tryβ¦except blocks swiftly debug your Python code, a program attempts to execute the code in a βtryβ block. If this fails, the βexceptβ block executes. The code in a βfinallyβ statement processes regardless of an βexceptβ block. These blocks are useful when youβre testing current code or creating new code. It makes sure that your program executes properly and does not contain any kind of errors.
Example :
def divide(a, b):
try:
result = a // b
except ZeroDivisionError:
print("No result, You are dividing by zero ")
else:
print("Your answer will be ", result)
finally:
print('Finally block is always executed')
divide(5, 4)
Output :
Your answer will be 1
Finally block is always executed
Python User-defined Exception
User-defined exception in python is one of the exceptions to treat the program in case it stops abruptly or something goes wrong. Python also provides an exception handling method with the help of try-except. Some of the standard exceptions which are most frequent include IndexError, ImportError, IOError, ZeroDivisionError exception, TypeError exception, and FileNotFoundError.
A Python program terminates as soon as it encounters an error. An error can be a syntax error or an exception in python. In this article, you will see what an exception is and how it differs from a syntax error. After that, you will learn about raising exceptions and making assertions. Then, youβll finish with a demonstration of the try and except block. A user-defined exception is in which the user can create his own error using the exception class.
How to create User-defined Exception
Users can specify their own exceptions by creating a fresh class called exception class. Exceptions need to be derive either directly or indirectly from the Exception class only. Below is a program to create a user-defined exception:
Example :
class DH(Exception):
# Constructor or Initializer
def __init__(self, value):
self.value = value
# __str__ is to print() the value
def __str__(self):
return(repr(self.value))
try:
raise(DH(10*2))
# Value of Exception is stored in error
except DH as error:
print('A New Exception occured: ',error.value)
Output :
A New Exception occured: 20
>
When the user is developing a vast program in python, it is a good practice to store all the raised programs in a separate file, especially user-defined exceptions. Many standard modules do this as they tend to define their exceptions separately as exceptions.py or errors.py
.
Creating user-defined exception using multiple inheritance
Exceptions from derived classed are created when a single module handles multiple several distinct errors. The user creates a base class for exceptions defined by that module. The user can inherit the base class by various user-defined class to handle different types of errors. User exception class can implement everything a normal class can do, but we generally make them simple and concise. Most implementations declare a custom base class and derive others exception classes from this base class