In this article, we will discuss about the null vs undefined vs undeclared variable in JavaScript. Sometimes we see these three words in our output, now letβs understand them one by one. But before going to further we must know what is variables and objects.
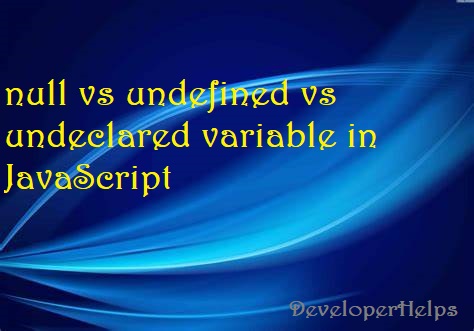
What is a variable in JavaScript
A variable in any programming language is named memory location which can be assigned a value. The value of a variable can be changed during the execution of the program.
What is an object in JavaScript
Object in JavaScript is also a type of variable but it contains multiple values. we can define and declare object in JavaScript with the following syntax:
let/const object_name = {
property:"value",
};
Example :
const student={
Name:"Ansh",
Roll_no:10,
}
Null in JavaScript
Null is a primitive data type in javascript. When a variable or object is declared with a null value that means it has no value at all. It represents an absence of a value.Β
An example with a null variable :
var car = null;
console.log(car);
Output :
null
An example with a null object :
let employee = {
Employee_id:null
};
console.log(employee.Employee_id);
Output:
null
Undefined in JavaScript
A variable or object is said to be undefined when it is declared but has not been assigned any value.
An example with variable :
var car;
console.log(car);
Output:
undefined
An example with object :
let employee = {
Employee_id,
};
console.log(employee.Employee_id);
Output:
Uncaught ReferenceError: Employee_id is not defined at <anonymous>:2:1
Note: null and undefined are loosely equal(==) but not strictly equal(===).
Undeclared in JavaScript
A variable or object is said to be undeclared when we use it prior to declaration.
An example with variable :
console.log(car);
Output :
uncaught reference error: car is not defined.
An example with object :
console.log(employee);
console.log(employee.Employee_id);
Output:
uncaught reference error: employee is not defined.
Note: When a variable is undeclared it will always throw an error.
Tip: For good practice, Never leave a variable undefined or undeclared. Always initialize them with any value or with a null value.