In this article, we will study Boolean in Javascript. Boolean is one of the data types of javascript that has true or false values. Boolean is used to specify whether the given condition is true or false.
Note: Except 0, NaN, and undefined all values are considered to be true. These values are supposed to be false always.

Boolean() function in JavaScript
The boolean () function returns the Boolean value (true or false) of a given variable, value, or expression.
Example 1:
Boolean(12)
Output :
true
Example 2:
a = 10
console.log(Boolean(a))
Output :
true
Example 3:
a = 0
console.log(Boolean(a))
Output :
false
Example 4:
console.log(Boolean(NaN))
Output :
false
We can also create a Boolean object of a value using a new operator
Boolean Object Syntax in JavaScript
var variable = new Boolean(value)
If you omit the value here then it will give the Boolean value ‘false’.
Example :
var a = new Boolean()
console.log(a)
var b = new Boolean('A')
console.log(b)
var c = new Boolean(10)
console.log(c)
var d = new Boolean(" ")
console.log(d)
var e = new Boolean(0)
console.log(e)
Output :
Boolean {false}
Boolean {true}
Boolean {true}
Boolean {true}
Boolean {false}
JavaScript Online Courses & Certification



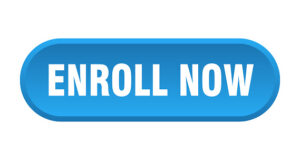
Comparison Operators and Conditions
Operator | Name | Example |
< | Less than | 12<19 (true) |
> | Greater than | 12>10(true) |
<= | Less than equal to | 12<=9 (false) |
>= | Greater than equal to | 12>=9 (true) |
== | Equal to | 12==12(true) |
!= | Not equal to | 12!=12(false) |
Comparison operators always return a Boolean value that is true or false. The condition to be made with the help of the comparison operator also returns true or false.
Example 1:
console.log(1>2)
Output :
false
Example 2:
console.log(1==1)
Output :
true
Example 3:
console.log(8>6)
Output :
true