Decorators are a powerful and intriguing feature in Python. Decorators are used to changing how a function or class behaves. In Decorators, functions are passed as arguments into another function. And weβll call this wrapped function inside the wrapper function.
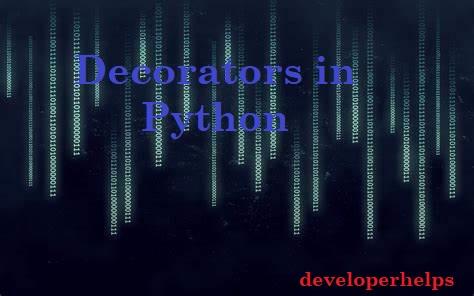
Decorators are nothing but a new way to call a function, or we can say they are used to decorate a called function. But before proceeding further in decorators, You should know about the functions in Python. You can learn about it here: function in Python.
Python Decorators Example
def smartfun(fun): #Step2
def inner(x,y): # step6
print("executing") # step7
fun(x,y) #Step8
return inner # step3
def add(a,b): # Step9
print(a+b)#step10
#function as arugument inside to another function
add =smartfun(add) #Step1 step4
add(8,4) # step5
Now, let us understand how this program will run :
Step 1: First our control goes to the βadd= smartfun(add)β line. Therefore, smartfun function is called with add argument.
Step 2: Control goes to the “smartfun(func)” function. And goes to the next step which is “def inner(x,y)” but this function will not execute because it is not called yet. So it will go to the last line which is
“return inner”. That means this function returns inner and is stored in add.
Step 3: Now control is in the “add(8,4)” line, as add function contains inner therefore it will call inner(x,y).
Step 4: Now our control is in inner(x,y) function and starts executing. (Here x contains 8 and y contains 4).
- First, it will print “executing”.
- Now come on to the next line which is “fun(x,y)”.Here you can see our fun is a function that you take as an argument in smartfun function or we can say it is a wrapped function add. In this line, it will call add (a,b) function.
Step 5: Now our control is in “def add(a, b)”, here a contains 8 and b contains 4. It will print a+b i.e. 12.
Output :
executing
12
This is a simple example of decorators and hopes you will understand the flow of decorator.
For using a decorator, we donβt need to write code the like the above. We can also use a short for which a decorator is designed.
Now let’s see the proper example of the decorator. The above example is only used to simplify the flow of the decorator.
Syntax of Decorator :
Except using this:
def add(a,b)
print(a+b)
add= smartfun(add)
We can simply use this:
@smartfun
def add(a,b):
print(a+b)
Both codes are equivalent to each other. So, with the exception of the first code, we will prefer the second one because it has fewer lines of code.
Example :
def inner(x,y):
print("executing")
fun(x,y)
return inner
@smartfun
def add(a,b):
print(a+b)
add(8,4)
Output :
executing
12