In this tutorial, we will know about the whole concept of Java String Replacement. First we have to know about String, A String can be defined as a sequence of Characters. Programming utilizes strings for the storage of text-based data. A wide range of characters, encompassing letters, numbers, symbols, and whitespaces, can be included in strings, with the possibility of zero or more characters forming them.
Within the realm of Java programming, strings are defined as a succession of characters confined within double quotes. A concrete illustration of a Java string is provided below:
String movie = “FastX”;
In this instance, the assignment of the string value “FastX” has been executed, allocating it to a variable referred to as “movie.”
Due to their capability for manipulation, strings serve as a valuable data type for developers in their interaction with text-based data. Java bestows upon strings an assortment of methods that permit their reversal, substitution, and general modification.
When confronted with the necessity of altering a particular character within a string and replacing it with an alternative character, the replace() method proves to be instrumental in accomplishing such a task.
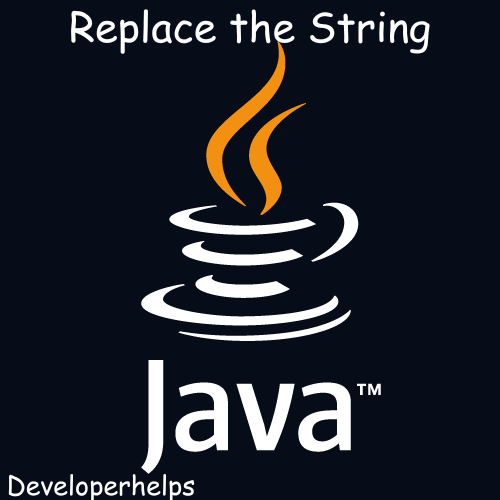
Java String Replacement Methods
- String replace(char oldChar, char newChar): The method “replace” is used to replace all occurrences of a specified character, represented by “oldChar,” with a new character or we can say replace character in string java , denoted by “newChar.” By invoking this method, a unique and elaborate transformation is applied to the original string. For instance, when the string “pog pance” is acted upon using the parameters (‘p’, ‘d’), a result of “dog dance” is obtained.
- String replaceFirst(String regex, String replacement) : The “replaceFirst” function, on the other hand, is designed to replace the initial substring that matches a defined regular expression, indicated by “regex,” with a replacement string. A distinctive and intricate operation is executed on the input string, where the first occurrence fitting the given pattern is substituted. However, if the specified regular expression is found to be invalid, a PatternSyntaxException is thrown, notifying the user of the error.
- String replaceAll(String regex, String replacement) : Lastly, the “replaceAll” method serves the purpose of replacing all substrings that conform to a provided regular expression, indicated by “regex,” with a replacement string. By utilizing this function, a remarkable and detailed transformation is performed on the input string, effectively substituting all occurrences that match the specified pattern.
Java replace() method
In the following example, the usage of the replace() method is being demonstrated with the string “str.” The occurrence of the character ‘o’ is being replaced with the character ‘p’ throughout the string. Similarly, in the second print statement, all instances of the character ‘i’ are being replaced with the character ‘K’.
The modified string is being showcased through the utilization of print statements; however, the original string “str” remains unaltered. To effectuate permanent changes, it is necessary to assign the returned string from the replace() method to a new string variable, allowing the modified string to retain the modifications permanently.
Syntax :
String replace(char oldChar, char newChar)
Example :
public class ReplaceMethodExample {
public static void main(String[] args) {
String str = "Fast and furious";
// Replacing 'o' with 'p'
String modifiedStr1 = str.replace('o', 'p');
// Replacing 'u' with 'K'
String modifiedStr2 = str.replace('u', 'K');
// Displaying the modified strings
System.out.println("Original string: " + str);
System.out.println("Modified string 1: " + modifiedStr1);
System.out.println("Modified string 2: " + modifiedStr2);
}
}
Output :
Original string: Fast and Furious
Modified string 1: Fast and Furipus
Modified string 2: Fast and FKrioKs
In this program, we have a string str
initialized with the value “Fast and Furious” We use the replace()
method to create two modified strings: modifiedStr1
and modifiedStr2
. The first modification replaces all occurrences of the character ‘o’ with ‘p’. While the second modification replaces all occurrences of the character ‘u’ with ‘K’. This is said to be java replace character in string.
The program then prints the original string followed by the two modified strings. As a result, we can observe the changes made by the replace()
method.
Java replaceFirst() Method
The use of the replaceFirst() method is being demonstrated in the following example. In this example, the part of a string is replaced with a new specified string or replace from string in java. A distinction can be made between the replaceFirst() and replaceAll() methods. While the replaceFirst() method replaces only the first occurrence, the replaceAll() method replaces all the occurrences.
Syntax :
String replaceFirst(String regex, String replacement);
Example :
public class ReplaceFirstExample {
public static void main(String[] args) {
String str = "Hello, World! Hello, World!";
// Replacing the first occurrence of "Hello" with "Hi"
String modifiedStr = str.replaceFirst("Hello", "Hi");
// Printing the original and modified strings
System.out.println("Original string: " + str);
System.out.println("Modified string: " + modifiedStr);
}
}
Output :
Original string: Hello, World! Hello, World!
Modified string: Hi, World! Hello, World!
In this program, the initial string str
contains the phrase “Hello, World! Hello, World!”. The replaceFirst()
method is used to replace the first occurrence of the substring “Hello” with “Hi”. The modified string is then stored in the variable modifiedStr
. Finally, both the original and modified strings are printed to the console, showcasing the changes made by the replaceFirst()
method.
Java replaceAll() Method
In the following example, the utilization of the replaceAll() method is demonstrated, whereby all instances of a designated substring are replaced with a new string.
The distinction between the replace() and replaceAll() methods lies in the fact that the replace() method carries out the replacement of all occurrences of an old character with a new character, whereas the replaceAll() method undertakes the replacement of all occurrences of an old string with the new string. Essentially, replace() is employed for character replacement, while replaceAll() is employed for partial string replacement.
Syntax :
String replaceAll(String regex, String replacement)
Example :
import java.util.Scanner;
import java.util.NoSuchElementException;
public class main{
public static void main(String args[]) {
try{
Scanner scanner = new Scanner(System.in);
System.out.println("Enter a sentence:");
String sentence = scanner.nextLine();
String modifiedSentence = sentence.replaceAll("cat", "dog");
System.out.println("Modified sentence:");
System.out.println(modifiedSentence);
scanner.close();
}catch (NoSuchElementException e) {
System.out.println("Type something in the Stdin box above.... and then Execute");
}
}
}
Output :
Enter a sentence:
I have a cat and my friend also has a cat.
Modified sentence:
I have a dog and my friend also has a dog.
In this program, the replaceAll()
method is used to replace all occurrences of the word “cat” with the word “dog” in the user’s inputted sentence. The modified sentence is then printed as the output. Note that the replaceAll()
method uses regular expressions to find and replace the matching patterns.
Thank you for reading our article about “Java String Replacement“. If you like our article, don’t forget to share it with your programmer friends.
Follow for More Info β https://instagram.com/developerhelps?igshid=MzRlODBiNWFlZA==